Tip on storing initial values in EEPROM of AVR microcontroller
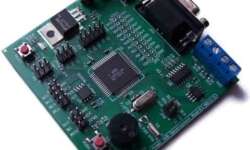
AVR microcontrollers have EEPROM memory built-in, which adds a lot of abilities to store constant values, calibration data, and other data that may be read and changed at any time during program flow. I have used EEPROM for storing the last device configuration on a couple of projects, e.g., in TDA7313 volume, BASS, TREBLE input, and output channel information is updated each time when it has been changed. After the device is turned ON, it reads the last saved settings from EEPROM memory and restores the last device state. But what happens when you turn the device for the first time. It has to read initial values that have to be stored or read ‘FF,’ which may lead to a crash or something else. One way is to prepare a separate *.eep file with initial EEPROM data and then burn it separately to microcontrollers EEPROM. But this may not always be handy. So this is a simple trick to store initial values to EEPROM at the first microcontroller run and skip this part later without additional files needing to be flashed in. Probably others are doing a bit. Differently, this is how I do. After main() I add following code:…