Compound interest is a concept in mathematics that many students find difficult to understand as compared to simple interest. If compound interest is your weak point too, then this article is for you. In this article, we will explain the compound interest in simple terms so that all your doubts related to the compound interest get clear. Also, you will learn to write Python code to find the compound interest. Python is an easy programming language, which is gaining popularity these days. If you are also learning Python or have a basic knowledge of the language, then it will be easy for you to understand this code. Read this and enhance your knowledge on Python with this code and get your compound interest related understanding.
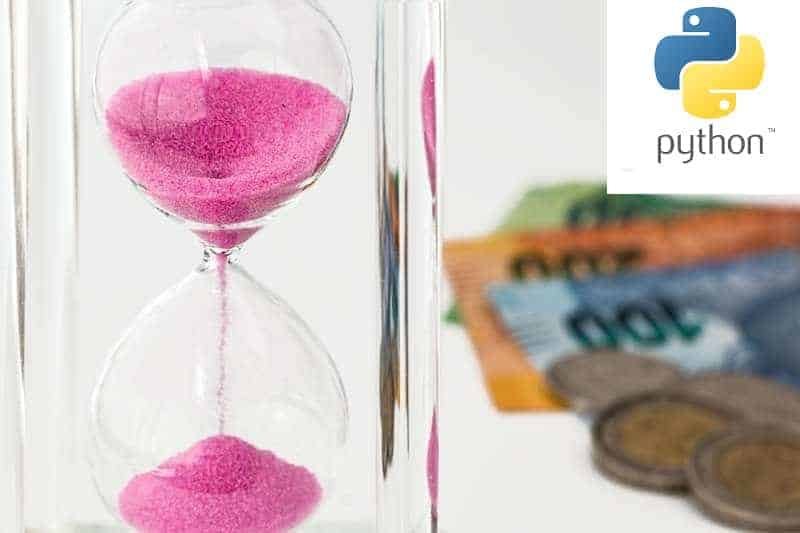
What Is Compound Interest?
Compound interest means gaining interest not only on the principal amount but gaining interest on the interest gained every year. In simple terms, we can say that compound interest is interest on interest. In compound interest, the Interest gained every year gets added back to the principal amount, so the principal amount changes. The time period after which the interest is added back to the principal amount varies as per the scenario. It can be annually, half-yearly, quarterly, or monthly. The shorter the period, the faster the principal amount grows.
Compound Interest Formula
There is a direct formula to calculate the compound interest. As per the formula, 1 is added to the ROI and is raised to the power the number of years. This is then multiplied with the principal amount. The principal amount is, then subtracted from the amount that comes after the calculation.
The formula for the compound interest (CI) is as:
where P is the initial invested amount or principal amount
r is the rate of interest
n is the time period for which interest gets compounded
t is the number of years for which the amount gets invested.
Here, n denotes the number of times the interest is compounded to the initial principal amount. So, we divide the rate by the number for which the interest is compounded in a year. As an example, if the interest is compounded annually, then n=1 and r is divided by 1. It is because the interest will be compounded once only in a year for the annual time period. Similarly, if the interest is to be compounded monthly, then the value of n here is 12, and the rate will be divided by 12 because the interest is compounded 12 times in a year.
Example of Compound Interest
Let us understand the compound interest with the help of this example. Consider that a person deposits Rs. 1500 in the bank, which is compounded bi-annually at the interest rate of 4.3% for six years. Here, in this case, the principal amount (P) is Rs. 1500, the ROI (r) is 0.043, the number of times the interest is compounded (n) is 2, and the number of years (t) is 6.
So, applying these values to the formula, we can calculate the compound interest.
Python code to calculate the Compound Interest
We can calculate the compound interest using the Python code. Before writing the python code, you should have a basic knowledge of Python and its syntax. Also, you should have an understanding of functions in Python. The command of these topics is a must to understand this code. Here you will see a program on how to calculate the compound interest.
Below is the Python code
# Function to calculate the Compound Interest
def comp_interest(p, r, t, n):
r=r/100
interest_amount = p * (pow(1+r/100n), n*t)
return interest_amount
# Prompts user to enter the input values
p=float(input(“Enter the principal (p) ”))
r=float(input(“Enter the rate of interest (r) ”))
n=float(input(“Enter the compounded time period (n) ”))
t=float(input(“Enter the number of years (t) ”))
# comp_interest() function is called
interest_val = comp_interest(p, r, t, n)
compound_interest = interest_val – p
#print the final result
print (“The compounded amount: “, interest_val)
print (“The compound interest: “, interest)
Output:
Enter the principal 100000
Enter the rate of interest 11
Enter the compounded time period 1
Enter the number of years 7
The compounded amount: 207616.02
The compound interest: 107616.02
Explanation of the above Python code
- In this program, the user gets prompted to enter the values of principal, rate of interest, number of years, and time compounded.
- The float() function converts the user input into float type. The values entered by the user get saved in variables p, r, n, and t, which are then passed to the function called comp_interest().
- This function calculates the values of the compounded amount, which gets saved in the variable called interest_amount.
- The function returns interest_amount that gets saved in the variable called interest_value. Using the value of p, and interest_value, the compound interest is calculated and stored in the variable called interest.
- Finally, the values of interest and compounded amount get printed.
Importance of Compound Interest Calculation Using Python
The calculation of Compound Interest gets complex sometimes and time-consuming too, based on the ROI. It is because the rate of interest is the power of an amount in the formula. So it is a little hard to calculate it manually. But Python code helps in the calculation fast. You usually have to enter the values of required parameters and run the code. The compound interest will get calculated automatically within no time.
How is Compound Interest Beneficial?
Compound Interest is beneficial because it returns the amount you invested along with the interest amount, which is large enough. Consider the below scenario to understand it well:
- Suppose Mr. A invested in some property, which returns around 9% on average every year. The amount he has invested is Rs. 50,000. So, here Rs. 50,000 is the principal amount for the first year.
- After one year, Mr. A gets his invested amount, i.e., Rs. 50,000 along with the interest amount of Rs. 4500.
- After two years, the amount becomes Rs. 59,405 (Rs. 54,500 + Rs. 54,500 * 9%).
- In this way, if Mr. A keeps his amount invested, then the gained value on this amount becomes quite high after ten years.
- However, the amount does not seem to be much higher than the invested amount, but the actual difference is visible in 30 to 40 years.
How Simple Interest Differs from Compound Interest?
Simple interest is different than a compound interest in the below terms:
- Simple interest is calculated simply based on a number of years, amount, and interest rate, while the compound interest is the interest on interest, which means it is calculated on the changing principal amount, which varies every year.
- Simple interest amount remains constant, whereas the compound interest, the interest is compounded every year.
- Simple interest is beneficial for the borrowers, while compound interest is beneficial for lenders.
Where Is the Compound Interest Used in Real Life?
The compound interest concept gets implemented on a few of the below real-life cases:
- Savings account: In a savings account, the quarterly interest is added in the case of some banks. It can get calculated using compound interest.
- Generating Profits: Compound Interest is a source of profits for businesses. The businesses need money to invest in the companies for which they take help from the investors. The companies try to please the investors by giving them dividends. If they invest the dividend again, then the profit will grow even faster.
- Credit cards: The credit card companies implement the compound interest on the outstanding amount if not paid on time. If the due amount is not paid on time, the interest on it keeps on getting accumulated. It keeps on increasing every month or in the cycle. That is why it is always suggested to pay the credit card dues on time.
- Pension Payments: The employers deduct some amount from the salaries of their employees as pension funds. This amount gets accumulated till the retirement age of the employer. The companies use the money in pension funds for investments that give the guarantee of returns. It helps them smoothen the pension payments cycle. Here, also the concept of the Compound Interest is used.
Conclusion
The article was all about Compound Interest and how to calculate the same using Python code. We hope the article was helpful to you in understanding the topic. You can find many similar articles on Cuemath that will make all your Mathematics related concepts clear. So learn commercial math with Cuemath and make Mathematics a fun subject. With the easy and simple ways to explain the mathematical concepts, be ready to top in Mathematics.