Program Flow In Embedded C
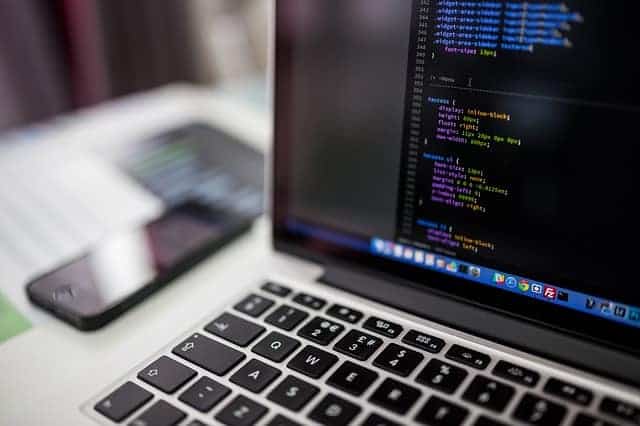
Program Flow and control is a control method of your program. For example, Loop constructions control repeated execution of repeated program segments where control is taken by control parameter. In this article, we will go through if/else switch/case statements and loop sentences like while, do while, for. While statement As I mentioned, three looping sentences available in C language one of them is While sentence. Let’s take an example: While(guess!=i) invokes looping operation. This causes the statement to be executed repeatedly at the beginning the condition guess!=i is checked. As long the argument is TRUE, the while sentence will be continued continuously. When guess becomes equal to i while statement will be skipped. I am not going too deep into it as there are tons of information about basic C.