Sometimes there is really a problem of how to generate truly random numbers using your microcontroller. Usually, a computer processor or any other MCU can generate a Pseudo-Random Number (PRN). These numbers are generated by algorithms, so-called Pseudo-Random Number Generators (PRNG). Everything that a pure algorithm produces is predictable at some level.
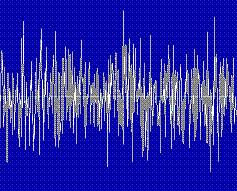
Many PRNG algorithms generate random numbers, but there is always a defined number of iterations when a random number sequence will repeat itself. Sometimes it may be acceptable. One popular way to generate pseudo-random numbers is using Timers. The universal algorithm is the concept of the Linear Feedback Shift Register (LFSR). LSFR is an n -bit register initiated with a non-zero seed value and is clocked by shifting values to the left and loading a new bit into bit0. The new bit is calculated by XORing the bits of selected taps of LSFR. This method is used in rand() functions.
Usually, we know the simple solution of random number generation (AVR-GCC example):
//Example how to generate PRN in range (0 to 9)
uint8_t randNumber;
// Get a random number (0 to 255)
randNumber = (uint8_t) rand();
// Set number range to 0 to 15
randNumber = randNumber & 0x0F;
// Set number range to 0 to 9
if (randNumber > 9)
randNumber -= 6;
But this algorithm will always get the same numerical order as long the same seed for the rand() function is used. This is nothing more than a mathematical function that cycles through a range of numbers that can be predictable. If you need really true randomness, you need to find a real-world source that could inject some entropy. This could be any noisy diode connected to ADC. Such random generators are so-called Hardware Random Number Generators. They often use some microscopic phenomena like thermal noise, photoelectric effect, etc. There are complete random number generators in the market connected to a PC via USB or another interface.
There is interesting reading about Random Noise Sources, whereas the entropy source is Zener diode used. Measurements are done using a PC Sound Card.
In general, if you are prototyping some Embedded platform with a temperature sensor like AD7416, this means that you already have a hardware random number generator. Because temperature sensor chips generate noise, which can be used as a source of entropy for your RNG. And you don’t need to connect additional devices like Zener diodes or photocells.
hallo..can I get an example source code for PRNG in microcontroller..thank’s