This is a simple demo program of reading button state, lighting LEDs, sending information via USART. 8 buttons are connected to Atmega16 port A, 8 LEDs to port B via current limiting resistors. While none of the buttons aren’t pressed, there is a running light on LEDs performed, but when any of the buttons are pressed, LEDs display the current 8 bit counter value in binary format. The same value is sent via USART – you can see a number in the terminal is connected.
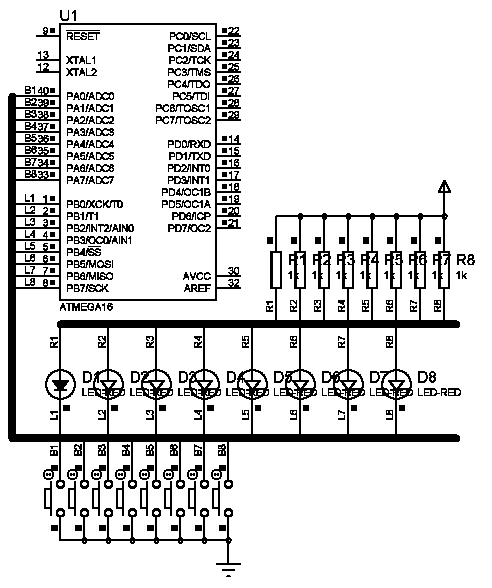
The program is very simple:
#include <avr/io.h>
#include <util/delay.h>
#include <stdio.h>
#ifndef F_CPU
#define F_CPU 1000000
#endif
//set desired baud rate
#define BAUDRATE 9600
//calculate UBRR value
#define UBRRVAL ((F_CPU/(BAUDRATE*16UL))-1)
char n, //number
c; //current state of display
int main(void)
{
//Set baud rate
UBRRL=UBRRVAL; //low byte
UBRRH=(UBRRVAL>>8); //high byte
//Set data frame format: asynchronous mode,no parity, 1 stop bit, 8 bit size
UCSRC=(1<<URSEL)|(0<<UMSEL)|(0<<UPM1)|(0<<UPM0)|
(0<<USBS)|(0<<UCSZ2)|(1<<UCSZ1)|(1<<UCSZ0);
//Enable Transmitter and Receiver
UCSRB=(1<<RXEN)|(1<<TXEN);
DDRA=0x00; //all A port pins as input
PORTA=0xFF; //Enable internal Pull-Up resistors
DDRB=0xFF; //All B port pins as output
while(1)
{
c=1; //start with first LED
while(PINA==0xFF) //wait for button to be pressed
{
if (c==0) c=1; //if light passed all leds start over
PORTB=~c; //LED on
_delay_ms(100);//short delay
n++; //increase number value
c=c<<1; //shift li9ghted LED
}
//wait for empty transmit buffer
while (!(UCSRA&(1<<UDRE))){};
UDR=n; //send number to USART
PORTB=~n; //and dislpaly number with led(binary)
_delay_ms(200); //long delay
PORTB=0xFF; //All LEDs OFF
}
return 0;
}
You can compile it with AVRStudio4. If you want to simulate the circuit by yourself, you can download the Proteus design file. The only limitation is that the Atmega16 model doesn’t support USART (only UART), so no terminal modeling is present.