Normally any embedded system has relations with the real world. The simplest and most common way is using buttons.
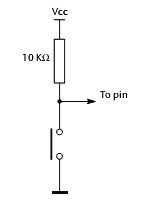
This is how applications interact with humans. But real-world like a human is not perfect. Practically mechanical contacts bounce (turns on and off repeatedly for a short period). And you cannot do anything about it. There is no need to look for higher quality buttons or try special button construction or even try to press the button precisely for simple applications. It is better to deal with it wisely. In digital circuits, there may be used special circuits like triggers, RC circuits, etc. But when working with microcontrollers, there is simpler to remove bouncing by adding a couple of code lines. Usually, switches bounce for less than 20ms; bigger ones may take up to 50ms.
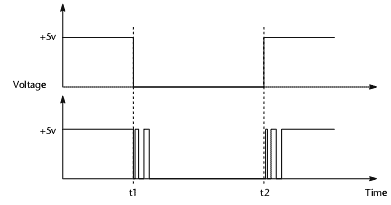
Buttons in embedded systems may cause a number of problems like:
- Rather reading value ‘A’ microcontroller may read ‘AAAAAA’;
- When the button is released, it bounces two – this may look like the button was pressed again after release.
To avoid this use simple routine:
- Read a pin;
- If the switch were detected – wait for 20ms and then reread pin;
- If the second reading confirms the first reading, then assume that button was pressed.
Select delay time according to buttons you use. If 20ms doesn’t guarantee stability, then add more ms. You may determine this time by using an oscilloscope.
https://scienceprog.com/dealing-with-switch-bounce-problem/
Using delays for debouncing is the simplest way but is not the most efficient!
Try this method and you’ll always use it!
#include
#define SW1 0 // PINB.0
#define DEBOUNCE_CNT 5 // Debounce Counter
unsigned char SW1Pressed (void) { // Checks if SW1 is pressed and debounced.
static unsigned char SW1KeyCount = 0, SW1KeyPressed = 0;
if (SW1KeyPressed) {
if (BITISSET(PINA,SW1)) {
if (SW1KeyCount < DEBOUNCE_CNT)
SW1KeyCount++;
else {
SW1KeyPressed = 0;
SW1KeyCount = 0;
}
}
} else {
if (BITISCLEAR(PINA,SW1)) {
if (SW1KeyCount < DEBOUNCE_CNT)
SW1KeyCount++;
else {
SW1KeyPressed = 1;
SW1KeyCount = 0;
return (1);
}
}
}
return (0);
}
int main(void) {
PORTB = (1 << SW1); // Internal Pull-Up
for(;;) {
if (SW1Pressed()) { // SW1 pressed and debounced
printf("SW1 Pressed.");
}
}
}
Using delays for debouncing is the simplest way but is not the most efficient!
Try this method and you’ll always use it!
#include
#define SW1 0 // PINB.0
#define DEBOUNCE_CNT 5 // Debounce Counter
unsigned char SW1Pressed (void) { // Checks if SW1 is pressed and debounced.
static unsigned char SW1KeyCount = 0, SW1KeyPressed = 0;
if (SW1KeyPressed) {
if (BITISSET(PINA,SW1)) {
if (SW1KeyCount < DEBOUNCE_CNT)
SW1KeyCount++;
else {
SW1KeyPressed = 0;
SW1KeyCount = 0;
}
}
} else {
if (BITISCLEAR(PINA,SW1)) {
if (SW1KeyCount < DEBOUNCE_CNT)
SW1KeyCount++;
else {
SW1KeyPressed = 1;
SW1KeyCount = 0;
return (1);
}
}
}
return (0);
}
int main(void) {
PORTB = (1 << SW1); // Internal Pull-Up
for(;;) {
if (SW1Pressed()) { // SW1 pressed and debounced
printf("SW1 Pressed.");
}
}
}
پروژه الکترونیک ARM STM32 LPC AVR PIC اتوماسیون مانیتورینگ کنترل صنعتی